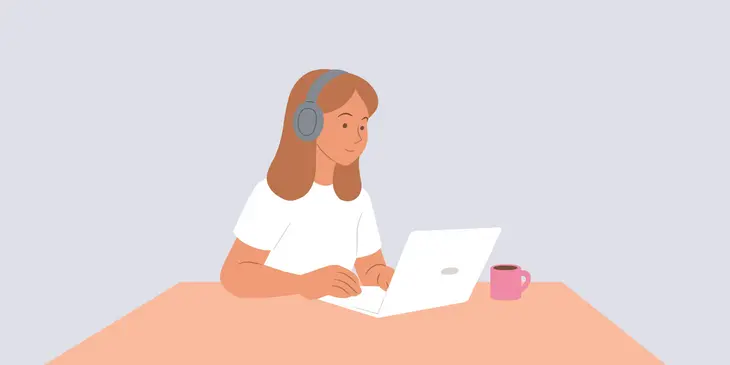
Python Best Practices: Writing Clean, Efficient, and Pythonic Code
- Admin
Python Best Practices: Writing Clean, Efficient, and Pythonic Code
Python is known for its readability, simplicity, and flexibility, but writing high-quality code requires more than just understanding the language syntax. In this article, we'll explore some best practices that will help you write clean, efficient, and Pythonic code that is easy to understand, maintain, and extend.
1. Follow PEP 8 Guidelines:
PEP 8 is the official style guide for Python code. Following PEP 8 guidelines ensures consistency and readability across your codebase. Pay attention to aspects such as naming conventions, indentation, spacing, and code layout. Using tools like linters and code formatters can help enforce PEP 8 compliance and catch potential issues early in the development process.
2. Write Readable Code:
Code is read more often than it is written. Write code that is easy to understand by using descriptive variable names, clear comments, and well-structured functions and classes. Aim for clarity and simplicity in your code, and avoid unnecessary complexity or clever tricks that can obscure its meaning.
3. Embrace Pythonic Idioms:
Python has its own set of idioms and conventions that experienced Python developers follow. Embrace these Pythonic idioms in your code, such as list comprehensions, generator expressions, context managers, and duck typing. Leveraging these idioms not only makes your code more concise and expressive but also improves its performance and readability.
4. Use Built-in Functions and Libraries:
Python comes with a rich standard library that provides a wide range of built-in functions and modules for common tasks. Whenever possible, use built-in functions and libraries instead of reinventing the wheel. Familiarize yourself with the standard library documentation and explore its offerings to find the right tools for your needs.
5. Handle Errors Gracefully:
Error handling is an essential aspect of writing robust and reliable code. Use try-except blocks to handle exceptions gracefully and provide informative error messages to users. Avoid catching generic exceptions and be specific about the types of errors you're handling. Additionally, use logging to record errors and debug information, making it easier to diagnose and fix issues in your code.
6. Write Unit Tests:
Unit testing is a crucial practice for ensuring the correctness and reliability of your code. Write unit tests for your functions and classes to validate their behavior under different conditions. Use testing frameworks like unittest or pytest to automate the testing process and run tests regularly as part of your development workflow.
7. Document Your Code:
Good documentation is essential for understanding how your code works and how to use it effectively. Write clear and concise docstrings for your functions, classes, and modules, following the numpydoc or Google-style docstring conventions. Additionally, provide high-level documentation and usage examples to help users understand the purpose and functionality of your code.
8. Refactor and Improve Continuously:
Code is never perfect, and there's always room for improvement. Regularly review and refactor your code to eliminate duplication, improve performance, and enhance readability. Solicit feedback from peers and incorporate code reviews into your development process to identify areas for improvement and learn from others' perspectives.
Conclusion:
By following these best practices, you can write clean, efficient, and Pythonic code that is easy to maintain, understand, and extend. Whether you're a beginner or an experienced developer, adopting these practices will help you become a better Python programmer and contribute to the overall quality of your codebase. Happy coding!
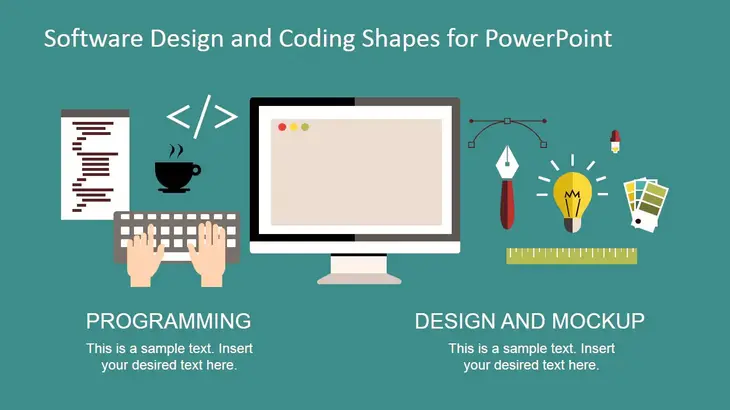